Pyomo.DoE: Optimization#
Our earlier exploratory analysis showed the sine wave experiment alone is rank deficient. What if instead of optimizing the sine wave parameters \(a\) and \(p\), we directly optimize \(u(t)\). In other words, we will formulate model-based design of experiments as an optimal control problem.
Maximize a scalar-valued function \(\psi(\cdot)\) of the Fisher information matrix \(\mathbf{M}\):
Pyomo.DoE
automatically formulates, initializes, and solves this optimization problem for \(\psi(\cdot) = \log_{10}(\mathrm{trace}(\cdot))\), i.e., A-optimality, and \(\psi(\cdot) = \log_{10}(\mathrm{det}(\cdot))\), i.e., D-optimality.
import sys
# If running on Google Colab, install Pyomo and Ipopt via IDAES
on_colab = "google.colab" in sys.modules
if on_colab:
!wget "https://raw.githubusercontent.com/dowlinglab/pyomo-doe/main/notebooks/tclab_pyomo.py"
# import TCLab model, simulation, and data analysis functions
from tclab_pyomo import (
TC_Lab_data,
TC_Lab_experiment,
extract_results,
extract_plot_results,
results_summary,
)
# set default number of states in the TCLab model
number_tclab_states = 2
Load experimental data (sine test)#
We will load the sine test data to serve as an initial point. Recall our create model function will use supplied data to initialize the Pyomo model. Carefully initialization is often required for optimization of large-scale dynamic systems.
import pandas as pd
if on_colab:
file = "https://raw.githubusercontent.com/dowlinglab/pyomo-doe/main/data/tclab_sine_test_5min_period.csv"
else:
file = '../data/tclab_sine_test_5min_period.csv'
df = pd.read_csv(file)
df.head()
Time | T1 | T2 | Q1 | Q2 | |
---|---|---|---|---|---|
0 | 0.00 | 22.2 | 22.2 | 50 | 0 |
1 | 1.01 | 22.2 | 22.2 | 51 | 0 |
2 | 2.01 | 22.2 | 22.2 | 52 | 0 |
3 | 3.00 | 22.2 | 22.2 | 53 | 0 |
4 | 4.01 | 22.2 | 22.2 | 54 | 0 |
For completeness, we will visualize the data again.
ax = df.plot(x='Time', y=['T1', 'T2'], xlabel='Time (s)', ylabel='Temperature (°C)')
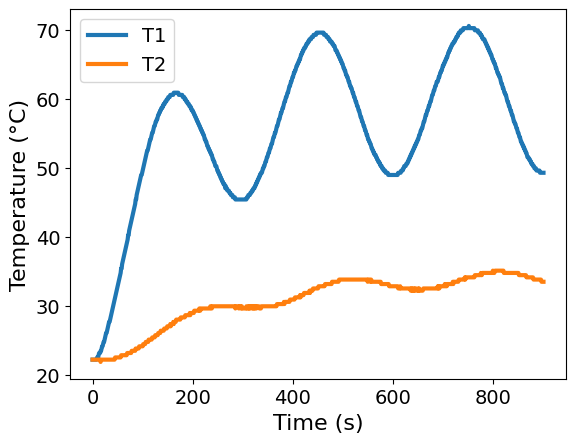
ax = df.plot(x='Time', y=['Q1', 'Q2'], xlabel='Time (s)', ylabel='Heater Power (%)')
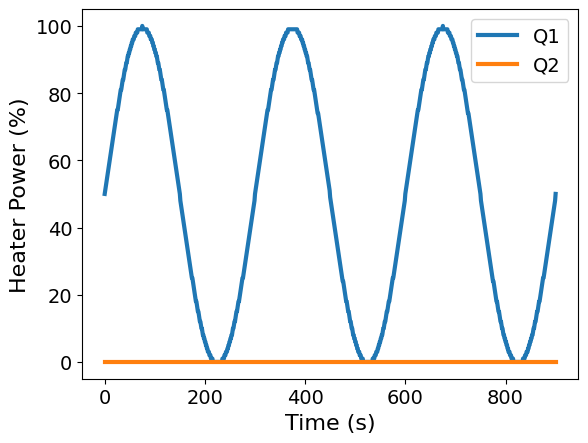
And then we will store the data in an instance of our TC_Lab_data
dataclass.
# Here, we will induce a step size of 6 seconds, as to not give too many
# degrees of freedom for experimental design.
skip = 6
# Create the data object considering the new control points every 6 seconds
tc_data = TC_Lab_data(
name="Sine Wave Test for Heater 1",
time=df['Time'].values[::skip],
T1=df['T1'].values[::skip],
u1=df['Q1'].values[::skip],
P1=200,
TS1_data=None,
T2=df['T2'].values[::skip],
u2=df['Q2'].values[::skip],
P2=200,
TS2_data=None,
Tamb=df['T1'].values[0],
)
Calculate FIM at initial point (sine test)#
We will start computing the FIM of the sine test experiment.
# Load Pyomo.DoE class
from pyomo.contrib.doe import DesignOfExperiments
from pyomo.environ import SolverFactory
# Copied from previous notebook
theta_values = {
'Ua': 0.0417051733576387,
'Ub': 0.009440714239773074,
'inv_CpH': 0.1659093525658045,
'inv_CpS': 5.8357556063605465,
}
# Create experiment object for design of experiments
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
# Create the design of experiments object using our experiment instance from above
TC_Lab_DoE = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
tee=True,)
FIM = TC_Lab_DoE.compute_FIM(method='sequential')
Finally, we will look at the eigendecomposition using the helper function results_summary
defined in tclab_pyomo.py
.
results_summary(FIM)
======Results Summary======
Four design criteria log10() value:
A-optimality: 5.773228885932493
D-optimality: 12.308607163946094
E-optimality: -1.793074158088514
Modified E-optimality: 7.514721752736364
FIM:
[[517225.40941304 1360.01262476 -66404.72541298 -1002.47319402]
[ 1360.01262476 5004.3737258 12379.2662576 5238.40389773]
[-66404.72541298 12379.2662576 65481.16908635 14190.01468139]
[ -1002.47319402 5238.40389773 14190.01468139 5526.94375493]]
eigenvalues:
[5.26802218e+05 6.26035823e+04 3.83207978e+03 1.61037063e-02]
eigenvectors:
[[-9.89752804e-01 -1.35949591e-01 4.36702406e-02 -7.52086327e-05]
[ 8.63262440e-04 -2.26164575e-01 -6.85698047e-01 -6.91857665e-01]
[ 1.42671125e-01 -9.31600001e-01 3.33329462e-01 -2.56487437e-02]
[ 5.79584008e-03 -2.49977462e-01 -6.45602485e-01 7.21578207e-01]]
Optimize next experiment (D-optimality)#
We are now ready to solve the optimization problem! Notice we create a new DesignOfExperiments
object and specify the prior_FIM
as result for the sine wave test. Thus we are decided what is the next best experiment to conduct. We already completed to sine test; we should use the data!
# Create experiment object for design of experiments
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
# Create the design of experiments object using our experiment instance from above
TC_Lab_DoE_D = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
objective_option="determinant", # Now we specify a type of objective, D-opt = "determinant"
prior_FIM=FIM, # We use the prior information from the existing experiment!
tee=True,)
TC_Lab_DoE_D.run_doe()
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1800
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 602
variables with only lower bounds: 0
variables with lower and upper bounds: 300
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.76e-01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 0.0000000e+00 3.55e-15 1.04e-02 -1.0 2.21e+00 - 9.11e-01 1.00e+00h 1
Number of Iterations....: 1
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 3.5527136788005009e-15 3.5527136788005009e-15
Number of objective function evaluations = 2
Number of objective gradient evaluations = 2
Number of equality constraint evaluations = 2
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 2
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 1
Total CPU secs in IPOPT (w/o function evaluations) = 0.002
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.41e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.98e-04 -1.0 3.89e-01 - 9.78e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 1.33e-03 -1.0 9.68e+00 - 9.40e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 5.56e-04 -1.7 3.29e+01 - 8.81e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 6.40e-18 -2.5 3.16e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 5.32e-19 -3.8 3.68e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 7.31e-21 -5.7 4.29e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.76e-15 3.85e-22 -8.6 6.23e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 3.8463898483715787e-22 3.8463898483715787e-22
Constraint violation....: 3.7608804959177178e-15 3.7608804959177178e-15
Complementarity.........: 5.3526636694796017e-09 5.3526636694796017e-09
Overall NLP error.......: 5.3526636694796017e-09 5.3526636694796017e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.006
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.41e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 6.51e-04 -1.0 5.04e-01 - 9.72e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 6.27e-04 -1.0 9.47e+00 - 9.75e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 9.84e-05 -1.7 6.36e+00 - 9.69e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.43e-05 -2.5 1.28e+01 - 9.59e-01 1.00e+00f 1
5 0.0000000e+00 3.55e-15 2.57e-19 -3.8 1.40e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 2.83e-20 -5.7 5.96e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.55e-15 1.41e-22 -8.6 4.56e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.4082749928715296e-22 1.4082749928715296e-22
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.7026222762342791e-09 4.7026222762342791e-09
Overall NLP error.......: 4.7026222762342791e-09 4.7026222762342791e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.016
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 4.62e-05 -1.0 1.03e-01 - 9.89e-01 1.00e+00f 1
2 0.0000000e+00 3.65e-15 2.43e-17 -1.0 9.93e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 5.33e-15 1.82e-17 -2.5 2.66e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 1.74e-08 -3.8 2.04e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.94e-08 -5.7 1.85e+00 - 9.96e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 4.73e-14 -8.6 3.85e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 4.7326499001393032e-14 4.7326499001393032e-14
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8319883555020095e-09 4.8319883555020095e-09
Overall NLP error.......: 4.8319883555020095e-09 4.8319883555020095e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.016
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.50e-05 -1.0 1.00e-01 - 9.90e-01 1.00e+00f 1
2 0.0000000e+00 3.80e-15 2.43e-17 -1.0 9.94e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.77e-17 -2.5 3.12e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 2.57e-07 -3.8 1.95e+00 - 9.97e-01 1.00e+00f 1
5 0.0000000e+00 3.77e-15 1.36e-08 -5.7 1.65e+00 - 9.97e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.44e-12 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.4351761207895442e-12 1.4351761207895442e-12
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.9764826151987226e-09 4.9764826151987226e-09
Overall NLP error.......: 4.9764826151987226e-09 4.9764826151987226e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.015
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.69e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 2.03e-04 -1.0 2.05e-01 - 9.83e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 8.33e-17 -1.0 9.63e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.37e-17 -1.7 1.29e+01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.52e-18 -2.5 8.06e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 4.40e-19 -3.8 2.21e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.91e-20 -5.7 4.75e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.55e-15 2.77e-22 -8.6 4.85e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.7710550520526427e-22 2.7710550520526427e-22
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8792798895046795e-09 4.8792798895046795e-09
Overall NLP error.......: 4.8792798895046795e-09 4.8792798895046795e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.016
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.69e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 1.71e-04 -1.0 2.41e-01 - 9.85e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 5.53e-04 -1.0 5.81e+00 - 9.54e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 4.71e-04 -1.7 4.04e+01 - 8.75e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 5.31e-18 -2.5 3.18e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 5.42e-19 -3.8 3.31e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 9.85e-21 -5.7 3.88e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 2.76e-15 2.34e-22 -8.6 5.49e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.3409211335250683e-22 2.3409211335250683e-22
Constraint violation....: 2.7616797737550769e-15 2.7616797737550769e-15
Complementarity.........: 5.1165889275871179e-09 5.1165889275871179e-09
Overall NLP error.......: 5.1165889275871179e-09 5.1165889275871179e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.016
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 4.84e-05 -1.0 1.05e-01 - 9.89e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 2.08e-17 -1.0 9.92e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.47e-17 -2.5 2.55e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 1.76e-19 -3.8 2.09e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.74e-08 -5.7 1.71e+00 - 9.96e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 2.26e-13 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.2587528186040976e-13 2.2587528186040976e-13
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8736431340332237e-09 4.8736431340332237e-09
Overall NLP error.......: 4.8736431340332237e-09 4.8736431340332237e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.015
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.51e-05 -1.0 1.00e-01 - 9.90e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 2.08e-17 -1.0 9.93e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.31e-17 -2.5 3.29e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.09e-07 -3.8 1.98e+00 - 9.96e-01 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.30e-08 -5.7 1.60e+00 - 9.97e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.66e-12 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.6640804565696806e-12 1.6640804565696806e-12
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.9986864279830130e-09 4.9986864279830130e-09
Overall NLP error.......: 4.9986864279830130e-09 4.9986864279830130e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 20744
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 1510
Total number of variables............................: 6487
variables with only lower bounds: 0
variables with lower and upper bounds: 3457
variables with only upper bounds: 0
Total number of equality constraints.................: 6487
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 5.54e+01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (330773)
1 0.0000000e+00 4.31e+05 9.28e-01 -1.0 3.58e+01 - 4.23e-01 1.00e+00h 1
2 0.0000000e+00 1.16e-10 5.50e-03 -1.0 4.31e+05 - 9.92e-01 1.00e+00h 1
Number of Iterations....: 2
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.1641532182693481e-10 1.1641532182693481e-10
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.1641532182693481e-10 1.1641532182693481e-10
Number of objective function evaluations = 3
Number of objective gradient evaluations = 3
Number of equality constraint evaluations = 3
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 3
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 2
Total CPU secs in IPOPT (w/o function evaluations) = 0.104
Total CPU secs in NLP function evaluations = 0.001
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 21992
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 1530
Reallocating memory for MA57: lfact (395831)
Total number of variables............................: 6648
variables with only lower bounds: 4
variables with lower and upper bounds: 3608
variables with only upper bounds: 0
Total number of equality constraints.................: 6497
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -1.3596140e+01 1.16e-10 3.35e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 -1.3594800e+01 7.48e-02 9.78e-03 -1.0 3.50e+02 - 9.90e-01 1.00e+00f 1
2 -1.3478717e+01 5.58e+02 2.19e-02 -1.0 3.02e+04 - 1.00e+00 1.00e+00f 1
3 -1.3012587e+01 7.38e+02 4.40e-01 -1.0 1.41e+05 - 1.00e+00 1.00e+00F 1
4 -1.3091923e+01 8.43e+01 1.23e-02 -1.0 9.36e+03 - 1.00e+00 1.00e+00h 1
5 -1.3090005e+01 1.35e-02 9.82e-05 -1.0 1.38e+02 - 1.00e+00 1.00e+00h 1
6 -1.3096572e+01 4.71e+00 1.77e-03 -2.5 1.90e+03 - 1.00e+00 1.00e+00h 1
7 -1.3354298e+01 6.94e+03 2.38e-01 -2.5 6.42e+04 - 1.00e+00 1.00e+00h 1
8 -1.3336877e+01 1.63e+02 1.03e-02 -2.5 1.72e+03 - 1.00e+00 1.00e+00h 1
9 -1.3335937e+01 2.25e+00 2.84e-05 -2.5 3.64e+01 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -1.3335958e+01 4.89e-05 2.94e-08 -2.5 1.23e+00 - 1.00e+00 1.00e+00h 1
11 -1.3554642e+01 4.41e+03 1.34e-01 -3.8 6.22e+04 - 8.17e-01 1.00e+00f 1
12 -1.3537124e+01 6.08e+01 3.08e-03 -3.8 2.39e+00 -4.0 1.00e+00 1.00e+00h 1
13 -1.3537055e+01 1.13e-01 2.83e-05 -3.8 6.18e-01 -4.5 1.00e+00 1.00e+00h 1
14 -1.3953722e+01 1.02e+04 3.01e-01 -3.8 3.28e+05 - 1.00e+00 6.55e-01F 1
15 -1.3934900e+01 5.53e+01 5.96e-03 -3.8 9.08e+00 -5.0 1.00e+00 1.00e+00h 1
16 -1.3934975e+01 5.19e+00 6.59e-05 -3.8 2.31e+00 -5.4 1.00e+00 1.00e+00h 1
17 -1.3935744e+01 2.89e+01 3.38e-05 -3.8 7.27e+00 -5.9 1.00e+00 1.00e+00h 1
18 -1.3938862e+01 2.27e+02 2.38e-04 -3.8 2.94e+01 -6.4 1.00e+00 1.00e+00h 1
19 -1.3950788e+01 3.53e+03 3.78e-03 -3.8 1.14e+02 -6.9 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -1.3974227e+01 5.77e+02 2.11e-03 -3.8 2.32e+02 -7.3 1.00e+00 1.00e+00h 1
21 -1.4153177e+01 5.16e+03 4.44e-02 -3.8 1.62e+05 - 4.54e-01 3.79e-01h 1
22 -1.4388656e+01 3.11e+03 5.58e-02 -3.8 7.86e+04 - 1.00e+00 9.88e-01h 1
23 -1.4333584e+01 4.91e+02 5.18e-03 -3.8 1.27e+04 - 1.00e+00 1.00e+00h 1
24 -1.4342298e+01 5.26e+02 3.99e-04 -3.8 4.26e+03 - 9.44e-01 9.31e-01h 1
25 -1.4341697e+01 1.11e+01 2.79e-05 -3.8 2.82e+01 -7.8 1.00e+00 1.00e+00h 1
26 -1.4343016e+01 1.29e+00 4.58e-06 -3.8 2.22e+02 - 1.00e+00 1.00e+00h 1
27 -1.4343013e+01 4.11e-02 3.03e-08 -3.8 2.07e+01 - 1.00e+00 1.00e+00h 1
28 -1.4343013e+01 3.47e-05 1.50e-09 -3.8 6.31e-01 - 1.00e+00 1.00e+00h 1
29 -1.4449436e+01 2.45e+03 7.92e-03 -5.7 1.66e+05 - 4.00e-01 4.95e-01f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -1.4487521e+01 2.12e+03 6.34e-03 -5.7 1.19e+05 - 2.72e-01 3.01e-01h 1
31 -1.4495022e+01 1.94e+03 5.79e-03 -5.7 7.35e+04 - 3.65e-01 9.17e-02h 1
32 -1.4498859e+01 8.40e+01 1.49e-04 -5.7 1.22e+02 -7.4 6.92e-01 1.00e+00h 1
33 -1.4503714e+01 8.38e+01 3.12e-04 -5.7 1.76e+05 - 1.10e-01 2.67e-02h 1
34 -1.4515382e+01 8.74e+01 2.95e-04 -5.7 1.37e+05 - 1.15e-01 7.83e-02h 1
35 -1.4519265e+01 1.10e+02 2.58e-04 -5.7 1.92e+05 - 1.04e-01 3.15e-02h 1
36 -1.4525395e+01 1.04e+02 2.55e-04 -5.7 7.36e+04 - 1.28e-01 7.34e-02h 1
37 -1.4532462e+01 9.72e+01 2.37e-04 -5.7 6.75e+04 - 1.49e-01 1.12e-01h 1
38 -1.4538538e+01 1.31e+02 2.03e-04 -5.7 9.53e+04 - 1.14e-01 1.30e-01h 1
39 -1.4540567e+01 1.47e+02 1.79e-04 -5.7 1.60e+05 - 1.24e-01 4.76e-02h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -1.4541394e+01 1.44e+02 1.91e-04 -5.7 5.19e+04 - 7.57e-02 2.85e-02h 1
41 -1.4543822e+01 1.32e+02 2.39e-04 -5.7 2.09e+04 - 3.29e-01 9.72e-02h 1
42 -1.4548414e+01 9.85e+01 4.46e-04 -5.7 2.42e+03 - 8.26e-01 2.61e-01h 1
43 -1.4552233e+01 4.34e+00 2.83e-04 -5.7 2.80e+02 -7.9 4.00e-01 1.00e+00h 1
44 -1.4553895e+01 4.38e+00 2.08e-04 -5.7 4.02e+02 -8.3 1.00e+00 3.44e-01h 1
45 -1.4555327e+01 3.39e+00 2.19e-04 -5.7 5.22e+02 -8.8 6.01e-01 3.27e-01f 1
46 -1.4558214e+01 1.53e+00 8.13e-06 -5.7 4.82e+02 -9.3 1.00e+00 1.00e+00h 1
47 -1.4558149e+01 5.09e+00 2.28e-04 -5.7 8.52e+02 -9.8 1.51e-01 4.75e-01H 1
48 -1.4557901e+01 4.77e+00 3.70e-06 -5.7 2.38e+02 -10.3 1.00e+00 1.00e+00f 1
49 -1.4558056e+01 3.28e+00 1.66e-05 -5.7 5.46e+02 -10.7 6.02e-01 6.92e-01H 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
50 -1.4558138e+01 1.17e+01 2.03e-04 -5.7 1.49e+04 -11.2 7.25e-01 3.25e-01h 1
51 -1.4558141e+01 1.15e+01 8.01e-05 -5.7 2.88e+02 -9.9 1.00e+00 1.81e-02h 1
52 -1.4558171e+01 2.35e+00 9.10e-04 -5.7 2.39e+03 - 4.09e-01 1.00e+00h 1
53 -1.4558281e+01 3.87e-01 1.05e-06 -5.7 8.97e+02 -10.4 1.00e+00 1.00e+00h 1
54 -1.4558432e+01 6.58e+00 2.22e-05 -5.7 3.78e+03 -10.8 9.53e-01 9.88e-01h 1
55 -1.4558443e+01 1.78e-02 1.88e-07 -5.7 1.17e+00 -6.8 1.00e+00 1.00e+00h 1
56 -1.4558458e+01 8.45e-02 1.23e-07 -5.7 2.30e+00 -7.3 1.00e+00 1.00e+00h 1
57 -1.4558462e+01 1.08e-01 6.33e-05 -5.7 9.97e+00 -7.7 1.00e+00 1.50e-01h 2
58 -1.4558491e+01 3.31e-02 4.07e-08 -5.7 6.85e+00 -8.2 1.00e+00 1.00e+00h 1
59 -1.4558496e+01 3.92e-03 4.25e-08 -5.7 2.15e+01 -8.7 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
60 -1.4558500e+01 3.31e-03 4.30e-08 -5.7 6.51e+01 -9.2 1.00e+00 1.00e+00h 1
61 -1.4558511e+01 2.78e-02 6.92e-08 -5.7 1.99e+02 -9.7 1.00e+00 1.00e+00h 1
62 -1.4558547e+01 2.72e-01 6.76e-07 -5.7 6.28e+02 -10.1 1.00e+00 1.00e+00h 1
63 -1.4558583e+01 4.54e-01 4.84e-05 -5.7 2.47e+04 - 1.44e-01 2.28e-02h 2
64 -1.4558618e+01 3.21e-01 1.88e-04 -5.7 1.31e+03 - 1.00e+00 4.40e-01h 1
65 -1.4558616e+01 6.26e-02 1.28e-07 -5.7 4.15e+02 - 1.00e+00 1.00e+00h 1
66 -1.4558617e+01 2.12e-06 1.87e-11 -5.7 5.78e-01 - 1.00e+00 1.00e+00h 1
67 -1.4560214e+01 3.61e-01 1.17e-05 -8.6 2.63e+02 - 7.17e-01 8.18e-01h 1
68 -1.4560565e+01 3.94e-02 2.70e-07 -8.6 2.22e+02 - 9.95e-01 9.80e-01h 1
69 -1.4560572e+01 1.32e-04 3.98e-10 -8.6 1.88e+01 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
70 -1.4560572e+01 2.33e-10 7.27e-14 -8.6 1.28e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 70
(scaled) (unscaled)
Objective...............: -1.4560571732274660e+01 -1.4560571732274660e+01
Dual infeasibility......: 7.2676893022525018e-14 7.2676893022525018e-14
Constraint violation....: 8.1238801465175856e-11 2.3283064365386963e-10
Complementarity.........: 2.5059035653642695e-09 2.5059035653642695e-09
Overall NLP error.......: 2.5059035653642695e-09 2.5059035653642695e-09
Number of objective function evaluations = 80
Number of objective gradient evaluations = 71
Number of equality constraint evaluations = 82
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 71
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 70
Total CPU secs in IPOPT (w/o function evaluations) = 2.831
Total CPU secs in NLP function evaluations = 0.032
EXIT: Optimal Solution Found.
Now let’s visualize the optimal experiment.
dopt_pyomo_doe_results = extract_plot_results(None, TC_Lab_DoE_D.model.scenario_blocks[0])
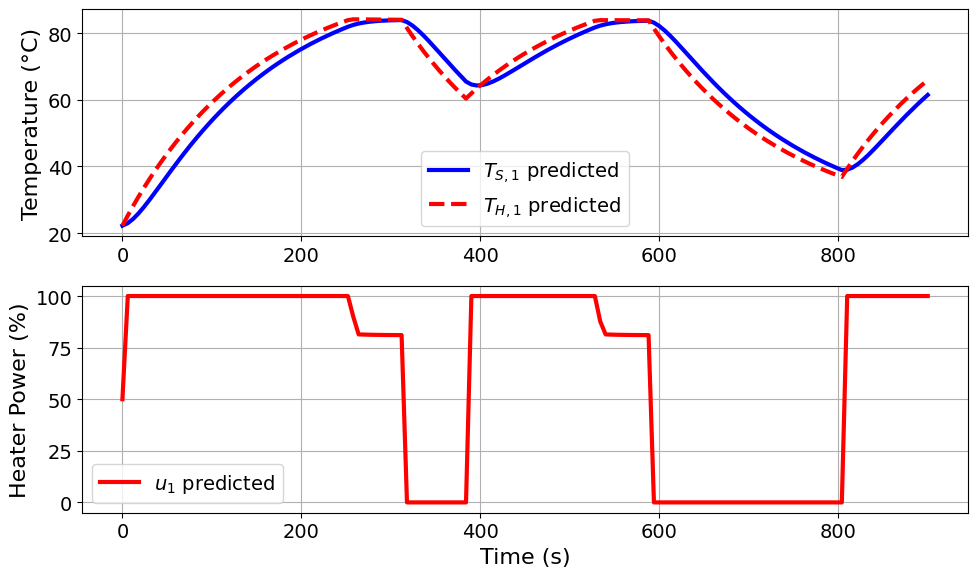
Model parameters:
Ua = 0.0421 Watts/degC
Ub = 0.0094 Watts/degC
CpH = 6.0274 Joules/degC
CpS = 0.1714 Joules/degC
Fascinating. The D-optimal experiment is close to a square wave that fluctuates between maximum power and off. The square wave transitions from full power to off when the predicted sensor temperature reaches ~85 °C. Likewise, the heater turns back on when the sensor cools to ~60 °C. Then the following cycle allows the sensor to return to 40 °C. This experiment captures two cooling cycles and almost three full heating events.
Finally, let’s analyze the predicted FIM of the new optimized experiment plus the existing sine wave experiment.
results_summary(TC_Lab_DoE_D.results['FIM'])
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.2351532377339645
D-optimality: 14.560571732277468
E-optimality: -0.9421764209340528
Modified E-optimality: 7.117191376655517
FIM:
[[1484716.4838525357, 8385.314034614812, -122009.42885862324, 3986.746701916149], [8385.314034614812, 12166.088352713867, 35185.570312046, 12908.796397561779], [-122009.42885862324, 35185.570312046, 207816.22848146953, 40882.82329357158], [3986.746701916149, 12908.796397561779, 40882.82329357158, 13815.84523653591]]
eigenvalues:
[1.49628718e+06 2.12204805e+05 1.00225443e+04 1.14241416e-01]
eigenvectors:
[[-9.95549809e-01 -8.97389772e-02 2.87661991e-02 9.14210614e-06]
[-3.39311263e-03 -1.85444140e-01 -6.95720553e-01 -6.93953795e-01]
[ 9.41756501e-02 -9.55575881e-01 2.78256029e-01 -2.40679407e-02]
[-1.09708330e-04 -2.10789286e-01 -6.61603356e-01 7.19617165e-01]]
Success. The FIM of the new optimized experiment and the existing sine wave experiment is not rank deficient! We see from the eigendecomposition we still have the least information about parameter \(C_p^S\). However, these results suggest we can uniquely estimate all four parameters using just two experiments.
Optimize next experiment (A-optimality)#
Next, we will consider A-optimality.
# Create experiment object for design of experiments
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
# Create the design of experiments object using our experiment instance from above
TC_Lab_DoE_A = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
objective_option="trace", # Now we specify a type of objective, A-opt = "trace"
prior_FIM=FIM, # We use the prior information from the same existing experiment as in the D-optimal case!
tee=True,)
TC_Lab_DoE_A.run_doe()
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1800
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 602
variables with only lower bounds: 0
variables with lower and upper bounds: 300
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.76e-01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 0.0000000e+00 3.55e-15 1.04e-02 -1.0 2.21e+00 - 9.11e-01 1.00e+00h 1
Number of Iterations....: 1
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 3.5527136788005009e-15 3.5527136788005009e-15
Number of objective function evaluations = 2
Number of objective gradient evaluations = 2
Number of equality constraint evaluations = 2
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 2
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 1
Total CPU secs in IPOPT (w/o function evaluations) = 0.002
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.41e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.98e-04 -1.0 3.89e-01 - 9.78e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 1.33e-03 -1.0 9.68e+00 - 9.40e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 5.56e-04 -1.7 3.29e+01 - 8.81e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 6.40e-18 -2.5 3.16e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 5.32e-19 -3.8 3.68e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 7.31e-21 -5.7 4.29e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.76e-15 3.85e-22 -8.6 6.23e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 3.8463898483715787e-22 3.8463898483715787e-22
Constraint violation....: 3.7608804959177178e-15 3.7608804959177178e-15
Complementarity.........: 5.3526636694796017e-09 5.3526636694796017e-09
Overall NLP error.......: 5.3526636694796017e-09 5.3526636694796017e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.009
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.41e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 6.51e-04 -1.0 5.04e-01 - 9.72e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 6.27e-04 -1.0 9.47e+00 - 9.75e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 9.84e-05 -1.7 6.36e+00 - 9.69e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.43e-05 -2.5 1.28e+01 - 9.59e-01 1.00e+00f 1
5 0.0000000e+00 3.55e-15 2.57e-19 -3.8 1.40e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 2.83e-20 -5.7 5.96e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.55e-15 1.41e-22 -8.6 4.56e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.4082749928715296e-22 1.4082749928715296e-22
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.7026222762342791e-09 4.7026222762342791e-09
Overall NLP error.......: 4.7026222762342791e-09 4.7026222762342791e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.010
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 4.62e-05 -1.0 1.03e-01 - 9.89e-01 1.00e+00f 1
2 0.0000000e+00 3.65e-15 2.43e-17 -1.0 9.93e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 5.33e-15 1.82e-17 -2.5 2.66e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 1.74e-08 -3.8 2.04e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.94e-08 -5.7 1.85e+00 - 9.96e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 4.73e-14 -8.6 3.85e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 4.7326499001393032e-14 4.7326499001393032e-14
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8319883555020095e-09 4.8319883555020095e-09
Overall NLP error.......: 4.8319883555020095e-09 4.8319883555020095e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.003
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.50e-05 -1.0 1.00e-01 - 9.90e-01 1.00e+00f 1
2 0.0000000e+00 3.80e-15 2.43e-17 -1.0 9.94e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.77e-17 -2.5 3.12e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 2.57e-07 -3.8 1.95e+00 - 9.97e-01 1.00e+00f 1
5 0.0000000e+00 3.77e-15 1.36e-08 -5.7 1.65e+00 - 9.97e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.44e-12 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.4351761207895442e-12 1.4351761207895442e-12
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.9764826151987226e-09 4.9764826151987226e-09
Overall NLP error.......: 4.9764826151987226e-09 4.9764826151987226e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.006
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.69e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 2.03e-04 -1.0 2.05e-01 - 9.83e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 8.33e-17 -1.0 9.63e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.37e-17 -1.7 1.29e+01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.52e-18 -2.5 8.06e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 4.40e-19 -3.8 2.21e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.91e-20 -5.7 4.75e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 3.55e-15 2.77e-22 -8.6 4.85e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.7710550520526427e-22 2.7710550520526427e-22
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8792798895046795e-09 4.8792798895046795e-09
Overall NLP error.......: 4.8792798895046795e-09 4.8792798895046795e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.008
Total CPU secs in NLP function evaluations = 0.001
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.69e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 1.71e-04 -1.0 2.41e-01 - 9.85e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 5.53e-04 -1.0 5.81e+00 - 9.54e-01 1.00e+00f 1
3 0.0000000e+00 3.55e-15 4.71e-04 -1.7 4.04e+01 - 8.75e-01 1.00e+00f 1
4 0.0000000e+00 3.55e-15 5.31e-18 -2.5 3.18e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 5.42e-19 -3.8 3.31e+00 - 1.00e+00 1.00e+00f 1
6 0.0000000e+00 3.55e-15 9.85e-21 -5.7 3.88e-01 - 1.00e+00 1.00e+00f 1
7 0.0000000e+00 2.76e-15 2.34e-22 -8.6 5.49e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 7
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.3409211335250683e-22 2.3409211335250683e-22
Constraint violation....: 2.7616797737550769e-15 2.7616797737550769e-15
Complementarity.........: 5.1165889275871179e-09 5.1165889275871179e-09
Overall NLP error.......: 5.1165889275871179e-09 5.1165889275871179e-09
Number of objective function evaluations = 8
Number of objective gradient evaluations = 8
Number of equality constraint evaluations = 8
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 8
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 7
Total CPU secs in IPOPT (w/o function evaluations) = 0.010
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 4.84e-05 -1.0 1.05e-01 - 9.89e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 2.08e-17 -1.0 9.92e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.47e-17 -2.5 2.55e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 1.76e-19 -3.8 2.09e+00 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.74e-08 -5.7 1.71e+00 - 9.96e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 2.26e-13 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 2.2587528186040976e-13 2.2587528186040976e-13
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.8736431340332237e-09 4.8736431340332237e-09
Overall NLP error.......: 4.8736431340332237e-09 4.8736431340332237e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.016
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1951
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 753
variables with only lower bounds: 0
variables with lower and upper bounds: 451
variables with only upper bounds: 0
Total number of equality constraints.................: 602
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 3.43e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (18093)
1 0.0000000e+00 3.55e-15 3.51e-05 -1.0 1.00e-01 - 9.90e-01 1.00e+00f 1
2 0.0000000e+00 3.55e-15 2.08e-17 -1.0 9.93e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 3.55e-15 1.31e-17 -2.5 3.29e+00 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 3.55e-15 3.09e-07 -3.8 1.98e+00 - 9.96e-01 1.00e+00f 1
5 0.0000000e+00 3.55e-15 1.30e-08 -5.7 1.60e+00 - 9.97e-01 1.00e+00f 1
6 0.0000000e+00 3.55e-15 1.66e-12 -8.6 3.95e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.6640804565696806e-12 1.6640804565696806e-12
Constraint violation....: 3.5527136788005009e-15 3.5527136788005009e-15
Complementarity.........: 4.9986864279830130e-09 4.9986864279830130e-09
Overall NLP error.......: 4.9986864279830130e-09 4.9986864279830130e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.008
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 20744
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 1510
Total number of variables............................: 6487
variables with only lower bounds: 0
variables with lower and upper bounds: 3457
variables with only upper bounds: 0
Total number of equality constraints.................: 6487
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 5.54e+01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (330773)
1 0.0000000e+00 4.31e+05 9.28e-01 -1.0 3.58e+01 - 4.23e-01 1.00e+00h 1
2 0.0000000e+00 1.16e-10 5.50e-03 -1.0 4.31e+05 - 9.92e-01 1.00e+00h 1
Number of Iterations....: 2
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.1641532182693481e-10 1.1641532182693481e-10
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.1641532182693481e-10 1.1641532182693481e-10
Number of objective function evaluations = 3
Number of objective gradient evaluations = 3
Number of equality constraint evaluations = 3
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 3
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 2
Total CPU secs in IPOPT (w/o function evaluations) = 0.099
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 21957
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 1511
Reallocating memory for MA57: lfact (395598)
Total number of variables............................: 6639
variables with only lower bounds: 1
variables with lower and upper bounds: 3608
variables with only upper bounds: 0
Total number of equality constraints.................: 6488
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -5.7732318e+00 4.78e+05 1.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 -5.8183289e+00 8.46e+04 1.38e+01 -1.0 3.78e+05 - 4.56e-01 1.00e+00h 1
2 -5.8492322e+00 2.91e+03 1.72e+00 -1.0 4.85e+04 - 8.36e-01 1.00e+00h 1
3 -5.8514044e+00 1.96e+00 3.18e-02 -1.0 3.54e+03 - 9.85e-01 1.00e+00h 1
4 -5.8761990e+00 5.55e+03 8.36e-03 -1.0 4.42e+04 - 9.35e-01 1.00e+00f 1
5 -5.9356123e+00 1.88e+04 1.88e-03 -1.0 1.10e+05 - 8.84e-01 1.00e+00h 1
6 -5.9517203e+00 7.35e+01 3.02e-06 -1.0 3.26e+04 - 1.00e+00 1.00e+00h 1
7 -5.9516866e+00 2.35e-02 1.00e-06 -1.0 7.72e+01 - 1.00e+00 1.00e+00h 1
8 -5.9519543e+00 2.37e-01 1.79e-07 -2.5 5.52e+02 - 1.00e+00 1.00e+00h 1
9 -5.9611275e+00 2.85e+02 1.07e-06 -2.5 1.91e+04 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -5.9613477e+00 3.09e-02 3.01e-08 -2.5 4.64e+02 - 1.00e+00 1.00e+00h 1
11 -5.9708350e+00 2.98e+02 3.56e-07 -3.8 2.02e+04 - 1.00e+00 1.00e+00h 1
12 -6.1437890e+00 7.30e+04 1.96e-04 -3.8 4.48e+05 - 1.00e+00 1.00e+00H 1
13 -6.1192787e+00 2.92e+03 1.29e-04 -3.8 7.64e+04 - 1.00e+00 1.00e+00h 1
14 -6.1281208e+00 1.99e+03 2.24e-06 -3.8 2.71e+04 - 1.00e+00 1.00e+00h 1
15 -6.1248634e+00 1.29e+02 5.06e-07 -3.8 1.00e+04 - 1.00e+00 1.00e+00h 1
16 -6.1250529e+00 1.64e-01 3.00e-08 -3.8 5.82e+02 - 1.00e+00 1.00e+00h 1
17 -6.1250389e+00 9.88e-04 1.50e-09 -3.8 4.29e+01 - 1.00e+00 1.00e+00h 1
18 -6.2287130e+00 3.96e+04 7.35e-06 -5.7 3.60e+05 - 7.91e-01 1.00e+00f 1
19 -6.3103098e+00 2.78e+04 3.99e-06 -5.7 4.06e+05 - 6.59e-01 8.62e-01h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -6.3248855e+00 1.04e+04 7.38e-06 -5.7 1.08e+05 - 6.23e-01 6.46e-01h 1
21 -6.3284025e+00 5.17e+03 6.84e-06 -5.7 3.36e+04 - 6.00e-01 5.11e-01h 1
22 -6.3284024e+00 4.21e+03 9.12e-03 -5.7 1.81e+01 -4.0 3.08e-04 1.86e-01h 1
23 -6.3294531e+00 1.06e+03 2.32e-03 -5.7 6.90e+03 - 1.00e+00 7.48e-01h 1
24 -6.3301892e+00 3.32e+02 7.15e-04 -5.7 5.25e+03 - 1.00e+00 6.90e-01h 1
25 -6.3305978e+00 2.69e+01 5.48e-05 -5.7 2.18e+03 - 1.00e+00 9.22e-01h 1
26 -6.3309354e+00 6.69e+01 1.56e-05 -5.7 4.30e+03 - 1.00e+00 7.12e-01h 1
27 -6.3310646e+00 8.46e+01 3.80e-06 -5.7 3.45e+03 - 1.00e+00 9.23e-01h 1
28 -6.3311316e+00 7.84e+01 2.86e-07 -5.7 2.91e+03 - 1.00e+00 1.00e+00h 1
29 -6.3311486e+00 4.37e+00 1.70e-08 -5.7 5.25e+02 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -6.3311476e+00 5.40e-01 2.98e-09 -5.7 2.10e+02 - 1.00e+00 1.00e+00h 1
31 -6.3311472e+00 6.84e-03 1.84e-11 -5.7 2.54e+01 - 1.00e+00 1.00e+00h 1
32 -6.3311472e+00 2.83e-08 2.52e-08 -5.7 7.56e-04 -4.5 1.00e+00 1.00e+00h 1
33 -6.3317543e+00 2.49e+01 1.64e-05 -8.6 1.04e+04 - 4.92e-01 2.90e-01f 1
34 -6.3320613e+00 8.32e+01 1.10e-05 -8.6 1.08e+04 - 1.96e-01 2.47e-01h 1
35 -6.3322159e+00 7.29e+01 1.09e-05 -8.6 3.95e+03 - 3.10e-01 2.00e-01h 1
36 -6.3322789e+00 6.62e+01 3.31e-05 -8.6 3.34e+03 - 3.02e-01 1.19e-01h 1
37 -6.3324274e+00 5.97e+01 2.28e-05 -8.6 3.07e+03 - 3.25e-01 3.19e-01h 1
38 -6.3325405e+00 3.85e+01 1.60e-05 -8.6 1.44e+03 - 5.61e-01 3.90e-01h 1
39 -6.3326164e+00 2.16e+01 8.84e-06 -8.6 8.18e+02 - 5.19e-01 4.60e-01h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -6.3326351e+00 1.69e+01 7.81e-06 -8.6 4.23e+02 - 5.87e-01 2.18e-01h 1
41 -6.3326729e+00 7.38e+00 8.30e-06 -8.6 3.28e+02 - 1.00e+00 5.71e-01h 1
42 -6.3326973e+00 8.31e-01 2.26e-06 -8.6 1.37e+02 - 1.00e+00 8.88e-01h 1
43 -6.3327003e+00 3.87e-05 3.36e-13 -8.6 1.48e+01 - 1.00e+00 1.00e+00h 1
Reallocating memory for MA57: lfact (422080)
44 -6.3327003e+00 1.23e-08 2.51e-14 -8.6 4.24e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 44
(scaled) (unscaled)
Objective...............: -6.3327003245850708e+00 -6.3327003245850708e+00
Dual infeasibility......: 2.5059035674162971e-14 2.5059035674162971e-14
Constraint violation....: 9.1604306362569332e-09 1.2289092410355805e-08
Complementarity.........: 2.7196815779234200e-09 2.7196815779234200e-09
Overall NLP error.......: 9.1604306362569332e-09 1.2289092410355805e-08
Number of objective function evaluations = 46
Number of objective gradient evaluations = 45
Number of equality constraint evaluations = 46
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 45
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 44
Total CPU secs in IPOPT (w/o function evaluations) = 1.596
Total CPU secs in NLP function evaluations = 0.005
EXIT: Optimal Solution Found.
dopt_pyomo_doe_results = extract_plot_results(None, TC_Lab_DoE_A.model.scenario_blocks[0])
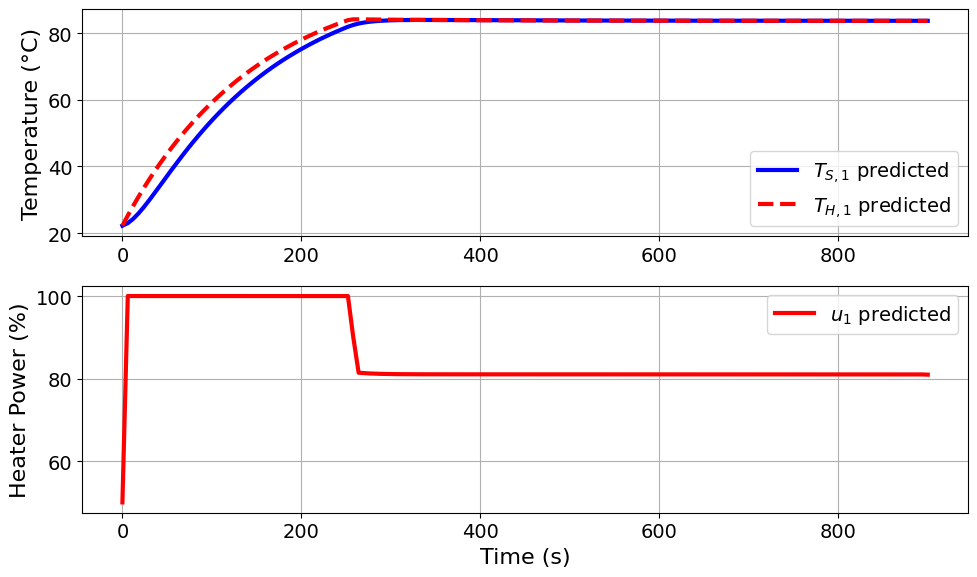
Model parameters:
Ua = 0.0421 Watts/degC
Ub = 0.0094 Watts/degC
CpH = 6.0274 Joules/degC
CpS = 0.1714 Joules/degC
Interestingly, we get a different optimal experiment. However, square-tooth like structure appears to emulate the first cycle in the D-optimal experiment, however achieving a higher temperature appears to be the best experiment for the A-optimal criteria.
results_summary(TC_Lab_DoE_A.results['FIM'])
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.332700324585071
D-optimality: 14.221895379167925
E-optimality: -1.035091770165665
Modified E-optimality: 7.3342634189119815
FIM:
[[1955865.1928081303, -10256.668510071942, -252788.48744997822, -18301.258965837907], [-10256.668510071942, 8250.475377585391, 27927.87862216795, 8884.520772699641], [-252788.48744997822, 27927.87862216795, 177519.01981971957, 32875.957483123544], [-18301.258965837907, 8884.520772699641, 32875.957483123544, 9662.079129546986]]
eigenvalues:
[1.99146028e+06 1.53941730e+05 5.89466513e+03 9.22376500e-02]
eigenvectors:
[[-9.90295022e-01 1.36479979e-01 -2.62484625e-02 -5.56588466e-05]
[ 7.12084874e-03 1.85358482e-01 6.96595323e-01 -6.93070330e-01]
[ 1.38323769e-01 9.50087656e-01 -2.78570003e-01 -2.44690500e-02]
[ 1.14716207e-02 2.10591771e-01 6.60654772e-01 7.20454565e-01]]
Sensitivity Analysis#
The eigendecomposition of the FIM above shows we have the least information (and thus greatest uncertainty) about parameter \(C_p^S\). In fact, notice that \(C_p^S\) goes to its bound in our earlier parameter estimation analysis.
This uncertainty motivates performing a sensivitiy analysis of the optimal experiment design to perturbations in \(C_p^S\). The code below focuses on A-optimality because it is computational less expensive, but and can easily adapted to D-optimality by changing the objective_option
.
import numpy as np
CpS_values = np.array([0.01, 0.05, 0.1, 0.5, 1.0])
a_opt = np.zeros((len(CpS_values)))
u_solutions = np.zeros((len(CpS_values), len(tc_data.time)))
Ts_solutions = np.zeros((len(CpS_values), len(tc_data.time)))
for i, v in enumerate(CpS_values):
print("\n********************\nCpS = ", v, " J/°C")
theta_values_new = theta_values.copy()
theta_values_new['inv_CpS'] = 1 / v
# Create experiment object for design of experiments
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values_new, number_of_states=number_tclab_states)
# Create the design of experiments object using our experiment instance from above
TC_Lab_DoE = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
tee=False,)
FIM_new = TC_Lab_DoE.compute_FIM(method='sequential')
# Create a new DoE object
TC_Lab_DoE = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
objective_option="trace", # We specify a type of objective, A-opt = "trace"
prior_FIM=FIM, # We use the prior information from the original experiment
tee=False,)
TC_Lab_DoE.run_doe()
pyomo_results = extract_results(TC_Lab_DoE.model.scenario_blocks[0])
results_summary(TC_Lab_DoE.results['FIM'])
a_opt[i] = np.log10(np.trace(TC_Lab_DoE.results['FIM']))
u_solutions[i, :] = pyomo_results.u1
Ts_solutions[i, :] = pyomo_results.TS1_data
print("********************\n")
********************
CpS = 0.01 J/°C
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.345620766498505
D-optimality: 16.557236420353764
E-optimality: 1.1762641874433832
Modified E-optimality: 5.1369934458645155
FIM:
[[2022826.6843716432, 829.8956823446237, -253458.792748775, -1844.3200988452588], [829.8956823446237, 5018.395139330466, 13267.86931681891, 5253.887159678284], [-253458.792748775, 13267.86931681891, 182871.0061206644, 15273.342611570326], [-1844.3200988452588, 5253.887159678284, 15273.342611570326, 5544.211892849836]]
eigenvalues:
[2.05711056e+06 1.51416060e+05 7.71867330e+03 1.50059739e+01]
eigenvectors:
[[-9.90975205e-01 -1.32948451e-01 1.71120584e-02 1.73845853e-04]
[ 4.70656783e-04 -9.34233205e-02 -6.91297021e-01 -7.16505611e-01]
[ 1.34031153e-01 -9.81169740e-01 1.38943069e-01 -6.03444276e-03]
[ 1.88989842e-03 -1.04416157e-01 -7.08879701e-01 6.97555205e-01]]
********************
********************
CpS = 0.05 J/°C
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.342325011779826
D-optimality: 16.321525241559577
E-optimality: 1.0002023526296024
Modified E-optimality: 5.309645954586698
FIM:
[[2006472.394538007, -1467.0401482268803, -253316.132823798, -5414.614507492659], [-1467.0401482268803, 5331.469940705959, 16889.27482496716, 5601.521979178391], [-253316.132823798, 16889.27482496716, 181767.3677006974, 19662.58659537228], [-5414.614507492659, 5601.521979178391, 19662.58659537228, 5934.062631847605]]
eigenvalues:
[2.04102492e+06 1.51702014e+05 6.76835666e+03 1.00046604e+01]
eigenvectors:
[[-9.90828557e-01 1.33692536e-01 -1.96231275e-02 9.89308405e-05]
[ 1.84539923e-03 1.16226648e-01 6.95096845e-01 -7.09456367e-01]
[ 1.35054779e-01 9.75416487e-01 -1.73837433e-01 -1.01700856e-02]
[ 3.94617173e-03 1.31073830e-01 6.97306143e-01 7.04675970e-01]]
********************
********************
CpS = 0.1 J/°C
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.338305077462897
D-optimality: 15.91622580578106
E-optimality: 0.6410420755686448
Modified E-optimality: 5.664468827976248
FIM:
[[1985809.1550228556, -4753.045405788305, -253116.64035824887, -10348.70079375541], [-4753.045405788305, 6221.3496716527125, 21476.075843530678, 6596.462411900629], [-253116.64035824887, 21476.075843530678, 180152.17262518042, 25177.03340049613], [-10348.70079375541, 6596.462411900629, 25177.03340049613, 7057.401320143186]]
eigenvalues:
[2.02074216e+06 1.52373364e+05 6.12017401e+03 4.37564495e+00]
eigenvectors:
[[-9.90627510e-01 1.34711035e-01 -2.25847797e-02 -1.64016116e-05]
[ 3.81334342e-03 1.45034949e-01 6.98333196e-01 -7.00914452e-01]
[ 1.36368025e-01 9.66326089e-01 -2.17618427e-01 -1.61205103e-02]
[ 6.80851583e-03 1.64413167e-01 6.81515146e-01 7.13063153e-01]]
********************
********************
CpS = 0.5 J/°C
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.308227354867407
D-optimality: 17.2140928274729
E-optimality: 1.743297276186294
Modified E-optimality: 4.5254408898399205
FIM:
[[1814818.2397931218, -46227.91439323254, -250460.46722158237, -66459.37899154567], [-46227.91439323254, 23869.06890217848, 52737.913904675544, 26917.54673919637], [-250460.46722158237, 52737.913904675544, 164083.29380926758, 62175.163641595085], [-66459.37899154567, 26917.54673919637, 62175.163641595085, 30650.63384980441]]
eigenvalues:
[1.85668473e+06 1.67154502e+05 9.52663022e+03 5.53729009e+01]
eigenvectors:
[[-0.98758136 0.1537699 -0.03203633 0.00339956]
[ 0.02979323 0.33411043 0.60897714 -0.71876938]
[ 0.14858665 0.84961147 -0.50532261 -0.02704482]
[ 0.04144194 0.37800184 0.61054859 0.69471404]]
********************
********************
CpS = 1.0 J/°C
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.2736109217669735
D-optimality: 17.87184745410738
E-optimality: 2.0770029315286553
Modified E-optimality: 4.146408066672371
FIM:
[[1604350.725326083, -117739.53034468756, -242878.6550187617, -155742.8293981735], [-117739.53034468756, 53729.0818745208, 74760.56129881516, 62446.54001365692], [-242878.6550187617, 74760.56129881516, 146437.60208523984, 88110.12839713677], [-155742.8293981735, 62446.54001365692, 88110.12839713677, 73116.50833673016]]
eigenvalues:
[1.67267281e+06 1.84654633e+05 2.01870712e+04 1.19399616e+02]
eigenvectors:
[[ 0.97679606 -0.21323534 0.01776713 0.00919096]
[-0.08283596 -0.45140663 -0.47514835 -0.75073584]
[-0.16570503 -0.70124636 0.69339326 0.00107751]
[-0.10746861 -0.50893642 -0.54140942 0.6605377 ]]
********************
Now let’s visualize how the A-optimality objective changes as a function of \(C_p^S\).
import matplotlib.pyplot as plt
plt.semilogx(CpS_values, a_opt, marker='o')
plt.xlabel('$C_p^S$ ( J / °C )')
plt.ylabel('log$_{10}$( trace(FIM) )')
plt.show()
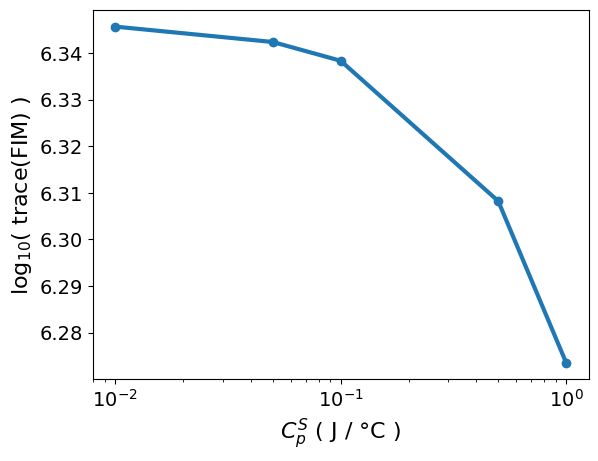
We see the objective changes insignificantly as we vary \(C_p^S\). What about the optimal solution?
for i, v in enumerate(CpS_values):
plt.plot(tc_data.time, u_solutions[i, :], label=f'$C_p^S$ = {v}')
plt.legend(ncol=2, loc='best')
plt.xlabel('Time (s)')
plt.ylabel('Heater Power (%)')
plt.show()
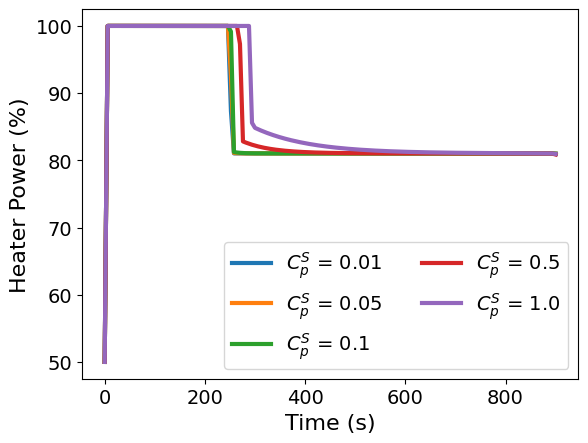
Interesting, the A-optimal next experiment does change on the assumed value of \(C_p^S\). For small values of \(C_p^S\), the optimal solutions are similar: start around \(u_1 = 100\)% and then decreases as the experiment progresses. As \(C_p^S\) increases, the length of time spent at 100% power increases.
Let’s also look at the optimal sensor temperature profile:
for i, v in enumerate(CpS_values):
plt.plot(tc_data.time, Ts_solutions[i, :], label=f'$C_p^S$ = {v}')
plt.legend(ncol=2, loc='best')
plt.xlabel('Time (s)')
plt.ylabel('Sensor Temperature (°C)')
plt.show()
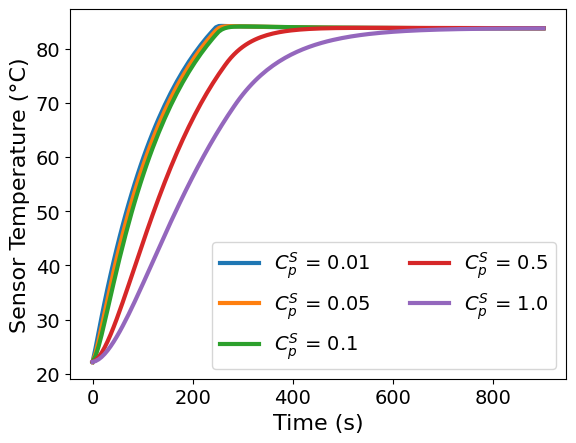
Now to be pragmatic. What should we do with this information? Here are some suggested next steps:
Confirm that these optimal experiments are all very informative for all of the values of \(C_p^S\). If this is the case, we can pick on, perform the experiment, and then refit our model.
Explore alternate formulations of MBDoE that consider uncertainty. We are working on a major overhaul of Pyomo.DoE that will make this much easier.