Exercise: Pyomo.DoE#
In this notebook, you will use Pyomo.DoE to compute the A- and D-optimal experiments from the TCLab. In our previous notebook, we used the sine test as a starting point. In this notebook, we will use the step test as the starting point.
Recall, we can computing the next best experiment assuming we already completed one prior experiment. Thus it is important to confirm our optimal experiment design does not change if we change the prior experiment of optimization initial point.
import sys
# If running on Google Colab, install Pyomo and Ipopt via IDAES
on_colab = "google.colab" in sys.modules
if on_colab:
!wget "https://raw.githubusercontent.com/dowlinglab/pyomo-doe/main/notebooks/tclab_pyomo.py"
else:
import os
if "exercise_solutions" in os.getcwd():
# Add the "notebooks" folder to the path
# This is needed for running the solutions from a separate folder
# You only need this if you run locally
sys.path.append('../notebooks')
# import TCLab model, simulation, and data analysis functions
from tclab_pyomo import (
TC_Lab_data,
TC_Lab_experiment,
extract_results,
extract_plot_results,
results_summary,
)
# set default number of states in the TCLab model
number_tclab_states = 2
Load and explore experimental data (step test)#
We will load the step test experimental data, similar to our previous notebooks.
import pandas as pd
if on_colab:
file = "https://raw.githubusercontent.com/dowlinglab/pyomo-doe/main/data/tclab_step_test.csv"
else:
file = '../data/tclab_step_test.csv'
df = pd.read_csv(file)
df.head()
Time | T1 | T2 | Q1 | Q2 | |
---|---|---|---|---|---|
0 | 0.00 | 22.84 | 22.84 | 50.0 | 0.0 |
1 | 1.00 | 22.84 | 22.84 | 50.0 | 0.0 |
2 | 2.01 | 23.16 | 22.84 | 50.0 | 0.0 |
3 | 3.02 | 22.84 | 22.84 | 50.0 | 0.0 |
4 | 4.01 | 22.84 | 22.84 | 50.0 | 0.0 |
ax = df.plot(x='Time', y=['T1', 'T2'], xlabel='Time (s)', ylabel='Temperature (°C)')
ax.grid(True)
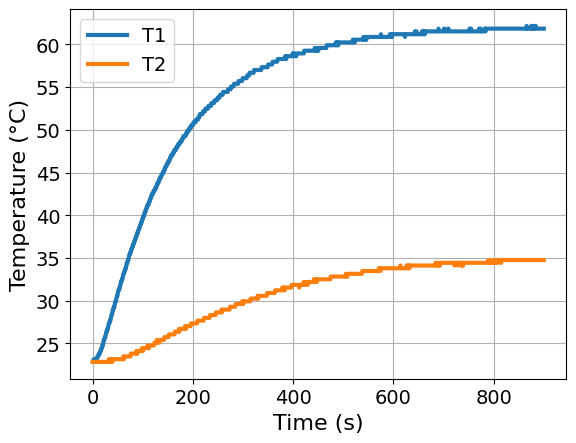
ax = df.plot(x='Time', y=['Q1', 'Q2'], xlabel='Time (s)', ylabel='Heater Power (%)')
ax.grid(True)
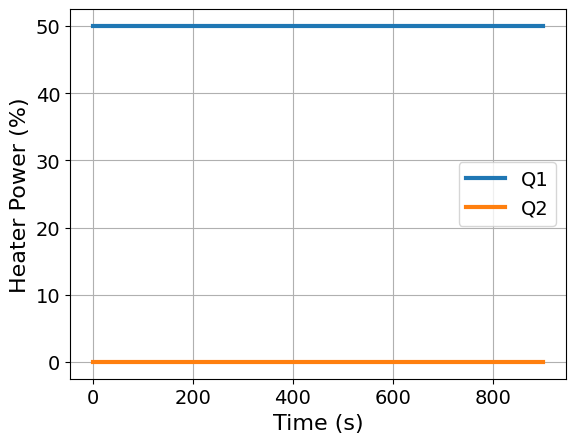
# Here, we will induce a step size of 10 seconds, as to not give too many
# degrees of freedom for experimental design.
skip = 10
# Create the data object considering the new control points every 6 seconds
tc_data = TC_Lab_data(
name="Sine Wave Test for Heater 1",
time=df['Time'].values[::skip],
T1=df['T1'].values[::skip],
u1=df['Q1'].values[::skip],
P1=200,
TS1_data=None,
T2=df['T2'].values[::skip],
u2=df['Q2'].values[::skip],
P2=200,
TS2_data=None,
Tamb=df['T1'].values[0],
)
Analyze FIM with Pyomo.DoE at initial point (step test)#
To get started, compute and analyze the FIM of the step test experiment.
# Load Pyomo.DoE class
from pyomo.contrib.doe import DesignOfExperiments
from pyomo.environ import SolverFactory
# Copied from previous notebook
theta_values = {
'Ua': 0.0417051733576387,
'Ub': 0.009440714239773074,
'inv_CpH': 0.1659093525658045,
'inv_CpS': 5.8357556063605465,
}
# Create experiment object for design of experiments
### BEGIN SOLUTION
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
### END SOLUTION
# Create the design of experiments object using our experiment instance from above
### BEGIN SOLUTION
TC_Lab_DoE = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
tee=True,)
### END SOLUTION
# Calculate the FIM
### BEGIN SOLUTION
FIM = TC_Lab_DoE.compute_FIM(method='sequential')
### END SOLUTION
# Call our custom function to summarize the results
# and compute the eigendecomposition of the FIM
### BEGIN SOLUTION
results_summary(FIM)
### END SOLUTION
======Results Summary======
Four design criteria log10() value:
A-optimality: 5.479278059341219
D-optimality: 8.809234454721581
E-optimality: -3.1126039391788907
Modified E-optimality: 8.570445782387669
FIM:
[[280055.18540639 -3320.41889892 -42508.35322699 -4661.44955393]
[ -3320.41889892 510.68043306 2729.38521901 584.8450854 ]
[-42508.35322699 2729.38521901 20251.11718816 3322.1354697 ]
[ -4661.44955393 584.8450854 3322.1354697 676.59046558]]
eigenvalues:
[2.86973532e+05 1.43167335e+04 2.03307130e+02 7.71606826e-04]
eigenvectors:
[[-9.87239625e-01 1.58273951e-01 -1.75293670e-02 1.33926737e-06]
[ 1.29823029e-02 1.58928598e-01 7.03772484e-01 -6.92298672e-01]
[ 1.57695528e-01 9.56659449e-01 -2.43536246e-01 -2.49983328e-02]
[ 1.79304963e-02 1.85724948e-01 6.67148491e-01 7.21177948e-01]]
Discussion: How does this FIM compare to the sine test experiment we previously analyzed?
Optimize the next experiment (A-optimality)#
Now we are ready to compute the A-optimal next best experiment. Why are we starting with A-optimality? It runs faster so it is better for debugging syntax.
# Create experiment object for design of experiments
### BEGIN SOLUTION
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
### END SOLUTION
# Create the design of experiments object using our experiment instance from above
### BEGIN SOLUTION
TC_Lab_DoE_A = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
objective_option="trace", # Now we specify a type of objective, A-opt = "trace"
prior_FIM=FIM, # We use the prior information from the same existing experiment as in the D-optimal case!
tee=True,)
### END SOLUTION
# Run DoE analysis
### BEGIN SOLUTION
TC_Lab_DoE_A.run_doe()
### END SOLUTION
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1080
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 362
variables with only lower bounds: 0
variables with lower and upper bounds: 180
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 0.0000000e+00 1.78e-15 5.10e-04 -1.0 5.18e-01 - 9.79e-01 1.00e+00h 1
Number of Iterations....: 1
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.7763568394002505e-15 1.7763568394002505e-15
Number of objective function evaluations = 2
Number of objective gradient evaluations = 2
Number of equality constraint evaluations = 2
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 2
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 1
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.94e-04 -1.0 2.57e-01 - 9.84e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.29e-17 -1.0 2.13e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 7.05e-18 -2.5 3.48e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.97e-19 -3.8 6.45e-01 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 1.80e-15 9.69e-21 -5.7 1.39e-01 - 1.00e+00 1.00e+00h 1
6 0.0000000e+00 1.39e-15 1.26e-22 -8.6 1.52e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2578315189634477e-22 1.2578315189634477e-22
Constraint violation....: 1.3877787807814457e-15 1.3877787807814457e-15
Complementarity.........: 3.2874888828090638e-09 3.2874888828090638e-09
Overall NLP error.......: 3.2874888828090638e-09 3.2874888828090638e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.006
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.22e-04 -1.0 3.32e-01 - 9.85e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.81e-17 -1.0 1.86e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 7.70e-18 -2.5 3.54e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.83e-19 -3.8 6.45e-01 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 1.78e-15 1.26e-20 -5.7 1.41e-01 - 1.00e+00 1.00e+00h 1
6 0.0000000e+00 1.78e-15 1.09e-22 -8.6 1.54e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.0867085297415401e-22 1.0867085297415401e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.3107750038258920e-09 3.3107750038258920e-09
Overall NLP error.......: 3.3107750038258920e-09 3.3107750038258920e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.99e-05 -1.0 3.56e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 6.07e-18 -1.7 6.32e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.86e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.26e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.29e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2862658525199580e-22 1.2862658525199580e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4533556448167006e-09 3.4533556448167006e-09
Overall NLP error.......: 3.4533556448167006e-09 3.4533556448167006e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.58e-05 -1.0 3.52e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.81e-18 -1.7 6.32e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.55e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.68e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.57e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.5695752123193700e-22 1.5695752123193700e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4533025836104998e-09 3.4533025836104998e-09
Overall NLP error.......: 3.4533025836104998e-09 3.4533025836104998e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 5.36e-05 -1.0 1.27e-01 - 9.89e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 2.69e-17 -1.7 5.40e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.69e-18 -3.8 1.04e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.24e-20 -5.7 1.70e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.18e-22 -8.6 1.84e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.1808003244194470e-22 1.1808003244194470e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4600225074837807e-09 3.4600225074837807e-09
Overall NLP error.......: 3.4600225074837807e-09 3.4600225074837807e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.004
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 4.90e-05 -1.0 1.18e-01 - 9.90e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 2.30e-17 -1.7 5.56e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.53e-18 -3.8 1.04e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.39e-20 -5.7 1.70e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.21e-22 -8.6 1.84e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2097516458588030e-22 1.2097516458588030e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4581298725181036e-09 3.4581298725181036e-09
Overall NLP error.......: 3.4581298725181036e-09 3.4581298725181036e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.99e-05 -1.0 3.64e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.86e-15 7.81e-18 -1.7 6.31e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.95e-15 1.46e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.18e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.57e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.5654393092566049e-22 1.5654393092566049e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4534443694469787e-09 3.4534443694469787e-09
Overall NLP error.......: 3.4534443694469787e-09 3.4534443694469787e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.58e-05 -1.0 3.47e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 2.66e-15 5.20e-18 -1.7 6.33e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.23e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.34e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.03e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.0288058868628282e-22 1.0288058868628282e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4532364509691435e-09 3.4532364509691435e-09
Overall NLP error.......: 3.4532364509691435e-09 3.4532364509691435e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.000
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 12464
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 910
Total number of variables............................: 3907
variables with only lower bounds: 0
variables with lower and upper bounds: 2077
variables with only upper bounds: 0
Total number of equality constraints.................: 3907
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.78e+01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (207913)
1 0.0000000e+00 2.54e+05 5.60e-03 -1.0 3.56e+01 - 9.35e-01 1.00e+00h 1
2 0.0000000e+00 1.16e-10 4.65e-12 -1.0 2.54e+05 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 2
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.1641532182693481e-10 1.1641532182693481e-10
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.1641532182693481e-10 1.1641532182693481e-10
Number of objective function evaluations = 3
Number of objective gradient evaluations = 3
Number of equality constraint evaluations = 3
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 3
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 2
Total CPU secs in IPOPT (w/o function evaluations) = 0.055
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 13197
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 911
Reallocating memory for MA57: lfact (249688)
Total number of variables............................: 3999
variables with only lower bounds: 1
variables with lower and upper bounds: 2168
variables with only upper bounds: 0
Total number of equality constraints.................: 3908
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -5.4792838e+00 2.72e+05 1.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (262838)
1 -5.5205848e+00 5.08e+04 1.26e+01 -1.0 2.25e+05 - 6.06e-01 1.00e+00h 1
2 -5.5604000e+00 1.72e+03 1.70e+00 -1.0 3.18e+04 - 9.03e-01 1.00e+00h 1
3 -5.5635707e+00 2.91e+00 2.92e-02 -1.0 2.66e+03 - 9.83e-01 1.00e+00h 1
4 -5.6121304e+00 8.19e+03 9.93e-04 -1.0 4.33e+04 - 8.92e-01 1.00e+00f 1
5 -5.6754752e+00 6.78e+03 1.73e-04 -1.0 6.43e+04 - 1.00e+00 1.00e+00h 1
6 -5.6790932e+00 2.37e+01 1.00e-06 -1.0 3.96e+03 - 1.00e+00 1.00e+00h 1
7 -5.6791515e+00 1.82e-03 1.00e-06 -1.0 6.41e+01 - 1.00e+00 1.00e+00h 1
8 -5.6796924e+00 4.74e-01 2.89e-07 -2.5 5.95e+02 - 1.00e+00 1.00e+00h 1
9 -5.6983805e+00 5.90e+02 5.33e-07 -2.5 2.10e+04 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -5.6988637e+00 1.41e-02 2.83e-08 -2.5 5.56e+02 - 1.00e+00 1.00e+00h 1
11 -5.7181112e+00 6.07e+02 1.16e-06 -3.8 2.27e+04 - 9.83e-01 1.00e+00h 1
12 -5.9507673e+00 6.34e+04 1.80e-03 -3.8 3.77e+05 - 1.00e+00 1.00e+00H 1
13 -5.9210919e+00 4.53e+03 1.33e-03 -3.8 5.90e+04 - 1.00e+00 1.00e+00h 1
14 -5.9211003e+00 3.50e+03 3.29e-02 -3.8 1.09e+02 -4.0 9.25e-01 2.41e-01h 1
15 -5.9121033e+00 2.55e+02 6.73e-03 -3.8 1.90e+04 - 1.00e+00 1.00e+00h 1
16 -5.9283851e+00 1.30e+03 3.46e-03 -3.8 6.43e+04 - 1.00e+00 4.85e-01h 2
17 -5.9364352e+00 2.17e+02 1.31e-06 -3.8 1.59e+04 - 1.00e+00 1.00e+00h 1
18 -5.9365759e+00 3.94e-01 1.88e-08 -3.8 2.80e+02 - 1.00e+00 1.00e+00h 1
19 -5.9365726e+00 1.32e-04 1.50e-09 -3.8 6.91e+00 - 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -6.0353683e+00 1.98e+04 1.01e-05 -5.7 2.21e+05 - 7.96e-01 1.00e+00f 1
21 -6.0734174e+00 8.35e+03 1.03e-05 -5.7 1.37e+05 - 5.97e-01 7.24e-01h 1
22 -6.0804794e+00 4.32e+03 7.64e-06 -5.7 3.92e+04 - 5.60e-01 4.95e-01h 1
23 -6.0822916e+00 2.97e+03 2.55e-05 -5.7 1.59e+04 - 5.48e-01 3.17e-01h 1
24 -6.0833848e+00 1.98e+03 5.06e-05 -5.7 9.15e+03 - 7.71e-01 3.33e-01h 1
25 -6.0833847e+00 1.63e+03 3.52e-03 -5.7 9.56e+00 -4.5 3.08e-03 1.78e-01h 1
26 -6.0842810e+00 5.47e+02 1.04e-03 -5.7 3.76e+03 - 2.47e-01 6.65e-01h 1
27 -6.0848595e+00 1.10e+02 2.07e-04 -5.7 2.02e+03 - 8.15e-01 8.01e-01h 1
28 -6.0851552e+00 2.12e+01 4.02e-05 -5.7 1.26e+03 - 1.00e+00 8.17e-01h 1
29 -6.0851552e+00 3.05e+00 1.93e-04 -5.7 1.23e+00 -5.0 9.06e-01 8.58e-01h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -6.0852583e+00 5.00e+00 1.29e-03 -5.7 8.81e+02 - 1.00e+00 6.24e-01h 1
31 -6.0853118e+00 2.33e+01 5.11e-05 -5.7 1.25e+03 - 1.00e+00 9.60e-01h 1
32 -6.0853456e+00 3.26e+01 9.11e-06 -5.7 1.19e+03 - 6.04e-01 1.00e+00h 1
33 -6.0853679e+00 1.69e+00 7.66e-11 -5.7 2.18e+02 - 1.00e+00 1.00e+00h 1
34 -6.0853866e+00 1.16e+00 9.63e-11 -5.7 2.46e+02 - 1.00e+00 1.00e+00h 1
35 -6.0853851e+00 3.15e-02 1.84e-11 -5.7 3.67e+01 - 1.00e+00 1.00e+00h 1
36 -6.0853851e+00 9.59e-07 1.84e-11 -5.7 2.11e-01 - 1.00e+00 1.00e+00h 1
37 -6.0857880e+00 1.26e+01 9.32e-06 -8.6 2.75e+03 - 5.49e-01 4.11e-01h 1
38 -6.0857883e+00 2.01e-02 2.57e-04 -8.6 2.94e+00 -5.4 1.83e-02 1.00e+00h 1
39 -6.0857896e+00 2.00e-02 2.78e-04 -8.6 1.11e+03 - 7.81e-01 3.27e-03h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -6.0860764e+00 3.60e-01 6.18e-05 -8.6 1.03e+03 - 9.44e-01 7.81e-01f 1
41 -6.0861453e+00 1.37e+00 1.33e-05 -8.6 2.47e+02 - 5.63e-01 7.84e-01h 1
42 -6.0861494e+00 1.47e+00 4.46e-05 -8.6 1.97e+03 - 1.00e+00 3.30e-02h 1
43 -6.0861639e+00 4.58e-02 7.89e-06 -8.6 4.20e+01 - 5.07e-01 9.69e-01h 1
44 -6.0861639e+00 7.87e-08 1.14e-07 -8.6 9.27e-02 -5.9 1.00e+00 1.00e+00h 1
45 -6.0861641e+00 6.45e-05 1.14e-05 -8.6 5.69e+00 - 3.99e-01 2.10e-01h 1
46 -6.0861641e+00 3.96e-07 4.75e-08 -8.6 1.15e-01 -6.4 1.00e+00 1.00e+00f 1
47 -6.0861646e+00 3.14e-03 2.77e-05 -8.6 6.97e+00 - 2.97e-01 1.00e+00h 1
48 -6.0861646e+00 7.42e-07 1.26e-08 -8.6 9.22e-02 -6.9 1.00e+00 1.00e+00h 1
49 -6.0861688e+00 2.02e+00 1.01e-05 -8.6 1.73e+02 - 5.17e-02 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
50 -6.0861700e+00 3.44e-06 1.47e-07 -8.6 3.22e+00 -7.3 1.00e+00 1.00e+00h 1
51 -6.0861703e+00 6.23e-05 1.57e-08 -8.6 1.03e+00 -7.8 1.00e+00 1.00e+00h 1
52 -6.0861704e+00 7.09e-04 1.66e-08 -8.6 3.26e+00 -8.3 1.00e+00 1.00e+00h 1
53 -6.0861708e+00 6.63e-03 1.69e-08 -8.6 9.95e+00 -8.8 1.00e+00 1.00e+00h 1
54 -6.0861722e+00 6.81e-02 1.80e-08 -8.6 3.19e+01 -9.2 1.00e+00 1.00e+00h 1
55 -6.0861763e+00 5.48e-01 1.84e-07 -8.6 1.18e+02 -9.7 1.00e+00 7.54e-01h 1
56 -6.0861745e+00 1.06e-01 2.77e-08 -8.6 3.98e+01 - 1.00e+00 1.00e+00h 1
57 -6.0861766e+00 1.14e-01 2.04e-08 -8.6 4.28e+01 - 1.00e+00 9.32e-01h 1
58 -6.0861767e+00 2.16e-07 4.66e-06 -8.6 2.09e-01 - 7.21e-01 1.00e+00h 1
59 -6.0861767e+00 1.82e-07 5.49e-08 -8.6 2.69e-02 - 9.95e-01 1.00e+00h 1
Reallocating memory for MA57: lfact (287702)
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
60 -6.0861767e+00 2.61e-08 2.51e-14 -8.6 9.61e-03 - 1.00e+00 1.00e+00h 1
61 -6.0861767e+00 9.17e-10 2.51e-14 -8.6 1.77e-03 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 61
(scaled) (unscaled)
Objective...............: -6.0861766779793482e+00 -6.0861766779793482e+00
Dual infeasibility......: 2.5059035581162194e-14 2.5059035581162194e-14
Constraint violation....: 9.0273285995244442e-10 9.1677065938711166e-10
Complementarity.........: 2.5190955416012936e-09 2.5190955416012936e-09
Overall NLP error.......: 2.5190955416012936e-09 2.5190955416012936e-09
Number of objective function evaluations = 66
Number of objective gradient evaluations = 62
Number of equality constraint evaluations = 66
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 62
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 61
Total CPU secs in IPOPT (w/o function evaluations) = 1.648
Total CPU secs in NLP function evaluations = 0.024
EXIT: Optimal Solution Found.
# Extract and plot the results using our custom function
### BEGIN SOLUTION
aopt_pyomo_doe_results = extract_plot_results(None, TC_Lab_DoE_A.model.scenario_blocks[0])
### END SOLUTION
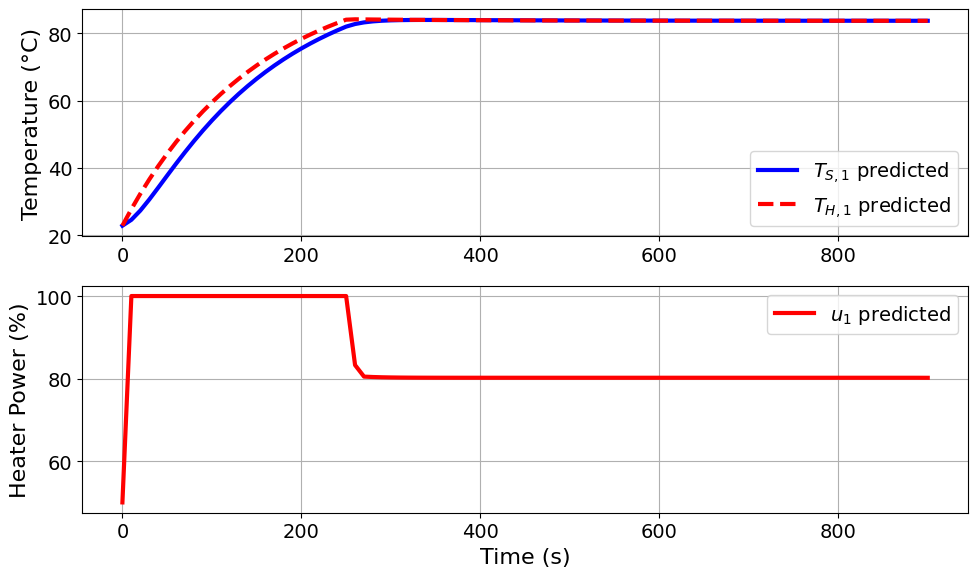
Model parameters:
Ua = 0.0421 Watts/degC
Ub = 0.0094 Watts/degC
CpH = 6.0274 Joules/degC
CpS = 0.1714 Joules/degC
# Compute the FIM at the optimal solution
### BEGIN SOLUTION
results_summary(TC_Lab_DoE_A.results['FIM'])
### END SOLUTION
======Results Summary======
Four design criteria log10() value:
A-optimality: 6.086176677979348
D-optimality: 12.10216308145059
E-optimality: -1.8430490851995411
Modified E-optimality: 7.903999360724161
FIM:
[[1128333.9541418809, -10344.660945130825, -152904.22848832433, -15046.559242758245], [-10344.660945130825, 2401.6027739254746, 11862.047413143466, 2711.232065796269], [-152904.22848832433, 11862.047413143466, 85659.76582339974, 14284.600287465535], [-15046.559242758245, 2711.232065796269, 14284.600287465535, 3090.281642352252]]
eigenvalues:
[1.15066864e+06 6.76851576e+04 1.13179676e+03 1.43532720e-02]
eigenvectors:
[[-9.89644319e-01 1.42450825e-01 -1.76602664e-02 9.29188738e-06]
[ 1.04215507e-02 1.59402422e-01 7.01400722e-01 -6.94636082e-01]
[ 1.42398295e-01 9.59088274e-01 -2.43539221e-01 -2.36866202e-02]
[ 1.47729345e-02 1.85602567e-01 6.69637059e-01 7.18971249e-01]]
Discussion: How do these compare to our previous A-optimal results considering the sine test as the prior experiment?
Optimize the next experiment (D-optimality)#
Finally, we are ready to solve the D-optimality problem. This may take 2 minutes to run.
# Create experiment object for design of experiments
### BEGIN SOLUTION
doe_experiment = TC_Lab_experiment(data=tc_data, theta_initial=theta_values, number_of_states=number_tclab_states)
### END SOLUTION
# Create the design of experiments object using our experiment instance from above
### BEGIN SOLUTION
TC_Lab_DoE_D = DesignOfExperiments(experiment=doe_experiment,
step=1e-2,
scale_constant_value=1,
scale_nominal_param_value=True,
objective_option="determinant", # Now we specify a type of objective, D-opt = "determinant"
prior_FIM=FIM, # We use the prior information from the existing experiment!
tee=True,)
### END SOLUTION
### BEGIN SOLUTION
TC_Lab_DoE_D.run_doe()
### END SOLUTION
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1080
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 362
variables with only lower bounds: 0
variables with lower and upper bounds: 180
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 0.0000000e+00 1.78e-15 5.10e-04 -1.0 5.18e-01 - 9.79e-01 1.00e+00h 1
Number of Iterations....: 1
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.7763568394002505e-15 1.7763568394002505e-15
Number of objective function evaluations = 2
Number of objective gradient evaluations = 2
Number of equality constraint evaluations = 2
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 2
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 1
Total CPU secs in IPOPT (w/o function evaluations) = 0.001
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.94e-04 -1.0 2.57e-01 - 9.84e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.29e-17 -1.0 2.13e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 7.05e-18 -2.5 3.48e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.97e-19 -3.8 6.45e-01 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 1.80e-15 9.69e-21 -5.7 1.39e-01 - 1.00e+00 1.00e+00h 1
6 0.0000000e+00 1.39e-15 1.26e-22 -8.6 1.52e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2578315189634477e-22 1.2578315189634477e-22
Constraint violation....: 1.3877787807814457e-15 1.3877787807814457e-15
Complementarity.........: 3.2874888828090638e-09 3.2874888828090638e-09
Overall NLP error.......: 3.2874888828090638e-09 3.2874888828090638e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.006
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.22e-04 -1.0 3.32e-01 - 9.85e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.81e-17 -1.0 1.86e+00 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 7.70e-18 -2.5 3.54e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.83e-19 -3.8 6.45e-01 - 1.00e+00 1.00e+00f 1
5 0.0000000e+00 1.78e-15 1.26e-20 -5.7 1.41e-01 - 1.00e+00 1.00e+00h 1
6 0.0000000e+00 1.78e-15 1.09e-22 -8.6 1.54e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 6
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.0867085297415401e-22 1.0867085297415401e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.3107750038258920e-09 3.3107750038258920e-09
Overall NLP error.......: 3.3107750038258920e-09 3.3107750038258920e-09
Number of objective function evaluations = 7
Number of objective gradient evaluations = 7
Number of equality constraint evaluations = 7
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 7
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 6
Total CPU secs in IPOPT (w/o function evaluations) = 0.006
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.99e-05 -1.0 3.56e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 6.07e-18 -1.7 6.32e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.86e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.26e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.29e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2862658525199580e-22 1.2862658525199580e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4533556448167006e-09 3.4533556448167006e-09
Overall NLP error.......: 3.4533556448167006e-09 3.4533556448167006e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.58e-05 -1.0 3.52e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 7.81e-18 -1.7 6.32e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.55e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.68e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.57e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.5695752123193700e-22 1.5695752123193700e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4533025836104998e-09 3.4533025836104998e-09
Overall NLP error.......: 3.4533025836104998e-09 3.4533025836104998e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 5.36e-05 -1.0 1.27e-01 - 9.89e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 2.69e-17 -1.7 5.40e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.69e-18 -3.8 1.04e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.24e-20 -5.7 1.70e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.18e-22 -8.6 1.84e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.1808003244194470e-22 1.1808003244194470e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4600225074837807e-09 3.4600225074837807e-09
Overall NLP error.......: 3.4600225074837807e-09 3.4600225074837807e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.65e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 4.90e-05 -1.0 1.18e-01 - 9.90e-01 1.00e+00h 1
2 0.0000000e+00 1.78e-15 2.30e-17 -1.7 5.56e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.53e-18 -3.8 1.04e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.39e-20 -5.7 1.70e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.21e-22 -8.6 1.84e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.2097516458588030e-22 1.2097516458588030e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4581298725181036e-09 3.4581298725181036e-09
Overall NLP error.......: 3.4581298725181036e-09 3.4581298725181036e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 1.99e-05 -1.0 3.64e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 1.86e-15 7.81e-18 -1.7 6.31e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.95e-15 1.46e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.18e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.57e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.5654393092566049e-22 1.5654393092566049e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4534443694469787e-09 3.4534443694469787e-09
Overall NLP error.......: 3.4534443694469787e-09 3.4534443694469787e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.005
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 1171
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 0
Total number of variables............................: 453
variables with only lower bounds: 0
variables with lower and upper bounds: 271
variables with only upper bounds: 0
Total number of equality constraints.................: 362
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 1.80e-03 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (11025)
1 0.0000000e+00 1.78e-15 2.58e-05 -1.0 3.47e-02 - 9.91e-01 1.00e+00h 1
2 0.0000000e+00 2.66e-15 5.20e-18 -1.7 6.33e-01 - 1.00e+00 1.00e+00f 1
3 0.0000000e+00 1.78e-15 1.23e-18 -3.8 1.03e-01 - 1.00e+00 1.00e+00f 1
4 0.0000000e+00 1.78e-15 1.34e-20 -5.7 1.68e-01 - 1.00e+00 1.00e+00h 1
5 0.0000000e+00 1.78e-15 1.03e-22 -8.6 1.83e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 5
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 1.0288058868628282e-22 1.0288058868628282e-22
Constraint violation....: 1.7763568394002505e-15 1.7763568394002505e-15
Complementarity.........: 3.4532364509691435e-09 3.4532364509691435e-09
Overall NLP error.......: 3.4532364509691435e-09 3.4532364509691435e-09
Number of objective function evaluations = 6
Number of objective gradient evaluations = 6
Number of equality constraint evaluations = 6
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 6
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 5
Total CPU secs in IPOPT (w/o function evaluations) = 0.015
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 12464
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 910
Total number of variables............................: 3907
variables with only lower bounds: 0
variables with lower and upper bounds: 2077
variables with only upper bounds: 0
Total number of equality constraints.................: 3907
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 0.0000000e+00 2.78e+01 0.00e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
Reallocating memory for MA57: lfact (207913)
1 0.0000000e+00 2.54e+05 5.60e-03 -1.0 3.56e+01 - 9.35e-01 1.00e+00h 1
2 0.0000000e+00 1.16e-10 4.65e-12 -1.0 2.54e+05 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 2
(scaled) (unscaled)
Objective...............: 0.0000000000000000e+00 0.0000000000000000e+00
Dual infeasibility......: 0.0000000000000000e+00 0.0000000000000000e+00
Constraint violation....: 1.1641532182693481e-10 1.1641532182693481e-10
Complementarity.........: 0.0000000000000000e+00 0.0000000000000000e+00
Overall NLP error.......: 1.1641532182693481e-10 1.1641532182693481e-10
Number of objective function evaluations = 3
Number of objective gradient evaluations = 3
Number of equality constraint evaluations = 3
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 3
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 2
Total CPU secs in IPOPT (w/o function evaluations) = 0.053
Total CPU secs in NLP function evaluations = 0.000
EXIT: Optimal Solution Found.
Ipopt 3.13.2: linear_solver=ma57
halt_on_ampl_error=yes
max_iter=3000
******************************************************************************
This program contains Ipopt, a library for large-scale nonlinear optimization.
Ipopt is released as open source code under the Eclipse Public License (EPL).
For more information visit http://projects.coin-or.org/Ipopt
This version of Ipopt was compiled from source code available at
https://github.com/IDAES/Ipopt as part of the Institute for the Design of
Advanced Energy Systems Process Systems Engineering Framework (IDAES PSE
Framework) Copyright (c) 2018-2019. See https://github.com/IDAES/idaes-pse.
This version of Ipopt was compiled using HSL, a collection of Fortran codes
for large-scale scientific computation. All technical papers, sales and
publicity material resulting from use of the HSL codes within IPOPT must
contain the following acknowledgement:
HSL, a collection of Fortran codes for large-scale scientific
computation. See http://www.hsl.rl.ac.uk.
******************************************************************************
This is Ipopt version 3.13.2, running with linear solver ma57.
Number of nonzeros in equality constraint Jacobian...: 13232
Number of nonzeros in inequality constraint Jacobian.: 0
Number of nonzeros in Lagrangian Hessian.............: 930
Reallocating memory for MA57: lfact (249922)
Total number of variables............................: 4008
variables with only lower bounds: 4
variables with lower and upper bounds: 2168
variables with only upper bounds: 0
Total number of equality constraints.................: 3917
Total number of inequality constraints...............: 0
inequality constraints with only lower bounds: 0
inequality constraints with lower and upper bounds: 0
inequality constraints with only upper bounds: 0
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
0 -1.0736863e+01 1.16e-10 7.55e+00 -1.0 0.00e+00 - 0.00e+00 0.00e+00 0
1 -1.0736366e+01 5.64e-02 1.09e-02 -1.0 2.33e+02 - 9.90e-01 1.00e+00f 1
2 -1.0694857e+01 3.87e+02 1.04e-02 -1.0 1.93e+04 - 1.00e+00 1.00e+00f 1
3 -1.0528361e+01 6.17e+03 1.76e-01 -1.0 7.36e+04 - 1.00e+00 1.00e+00h 1
4 -1.0540616e+01 6.68e+00 1.21e-03 -1.0 6.09e+03 - 1.00e+00 1.00e+00h 1
5 -1.0567725e+01 1.33e+01 1.40e-02 -1.7 2.28e+03 - 1.00e+00 1.00e+00h 1
6 -1.0678122e+01 2.09e+02 5.07e-02 -1.7 8.83e+03 - 1.00e+00 1.00e+00h 1
7 -1.0678363e+01 8.88e-02 1.30e-04 -1.7 1.33e+02 - 1.00e+00 1.00e+00h 1
8 -1.0821350e+01 3.45e+02 9.07e-02 -2.5 1.18e+04 - 9.94e-01 1.00e+00h 1
9 -1.0815878e+01 2.00e+00 6.97e-04 -2.5 1.06e+00 -4.0 1.00e+00 1.00e+00h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
10 -1.1149998e+01 2.83e+03 3.65e-01 -2.5 4.32e+04 - 1.00e+00 1.00e+00h 1
11 -1.1123669e+01 1.97e+01 9.17e-03 -2.5 2.38e+00 -4.5 1.00e+00 1.00e+00h 1
12 -1.1262302e+01 3.68e+02 5.38e-02 -2.5 6.86e+03 - 1.00e+00 1.00e+00h 1
13 -1.1266670e+01 3.66e+02 5.29e-02 -2.5 8.86e+03 - 3.80e-01 1.79e-02h 5
14 -1.1261373e+01 5.51e+00 6.69e-04 -2.5 1.35e+00 -5.0 1.00e+00 1.00e+00h 1
15 -1.1342731e+01 2.49e+01 2.02e-02 -2.5 1.90e+03 - 1.00e+00 1.00e+00H 1
16 -1.1351632e+01 2.25e+01 3.55e-04 -2.5 3.08e+02 - 1.00e+00 1.00e+00h 1
17 -1.1351558e+01 4.46e-01 5.84e-06 -2.5 6.80e+01 - 1.00e+00 1.00e+00h 1
18 -1.1351567e+01 1.39e-05 2.83e-08 -2.5 8.47e-01 - 1.00e+00 1.00e+00h 1
19 -1.1789730e+01 3.78e+03 4.15e-01 -3.8 5.30e+04 - 6.12e-01 1.00e+00f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
20 -1.2120142e+01 4.73e+03 1.99e-01 -3.8 8.96e+04 - 6.46e-01 6.33e-01h 1
21 -1.2316219e+01 4.19e+03 1.05e-01 -3.8 9.60e+04 - 5.04e-01 5.83e-01h 1
22 -1.2294937e+01 5.53e+01 2.94e-03 -3.8 1.03e+01 -5.4 1.00e+00 1.00e+00h 1
23 -1.2486000e+01 2.11e+03 3.47e-02 -3.8 1.04e+05 - 5.98e-01 5.53e-01h 1
24 -1.2499469e+01 1.21e+03 1.42e-02 -3.8 3.65e+04 - 7.33e-01 5.90e-01h 1
25 -1.2503729e+01 1.88e+01 2.39e-04 -3.8 6.46e+03 - 1.00e+00 1.00e+00h 1
26 -1.2504801e+01 1.03e+00 4.60e-06 -3.8 7.78e+02 - 1.00e+00 1.00e+00h 1
27 -1.2505229e+01 8.56e-03 9.85e-06 -3.8 1.11e+03 - 1.00e+00 1.00e+00H 1
28 -1.2505145e+01 3.20e-05 3.49e-08 -3.8 5.71e+01 - 1.00e+00 1.00e+00H 1
29 -1.2548502e+01 2.04e+02 1.30e-03 -5.7 5.97e+04 - 3.37e-01 3.23e-01f 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
30 -1.2550590e+01 2.23e+02 1.42e-03 -5.7 7.73e+05 - 1.41e-02 5.55e-03h 1
31 -1.2552042e+01 9.65e-01 2.56e-04 -5.7 1.44e+01 -5.9 5.67e-01 1.00e+00h 1
32 -1.2552612e+01 9.68e-01 2.97e-04 -5.7 6.68e+04 - 1.40e-01 3.46e-03h 1
33 -1.2573342e+01 3.88e+01 4.29e-04 -5.7 5.88e+04 - 2.12e-01 1.49e-01f 1
34 -1.2584389e+01 4.80e+01 4.44e-04 -5.7 4.34e+04 - 2.18e-01 1.23e-01h 1
35 -1.2591849e+01 8.81e+01 5.49e-04 -5.7 7.39e+04 - 3.17e-01 9.98e-02h 1
36 -1.2604297e+01 7.52e+01 4.61e-04 -5.7 1.48e+04 - 3.51e-01 2.70e-01h 1
37 -1.2607331e+01 6.59e+01 5.56e-04 -5.7 1.72e+03 - 3.36e-01 1.26e-01h 1
38 -1.2613652e+01 4.54e+01 5.69e-04 -5.7 1.56e+03 - 5.59e-01 3.28e-01h 1
39 -1.2625245e+01 6.37e+00 9.36e-05 -5.7 8.64e+02 - 1.00e+00 9.83e-01h 1
iter objective inf_pr inf_du lg(mu) ||d|| lg(rg) alpha_du alpha_pr ls
40 -1.2624822e+01 6.53e-02 4.15e-07 -5.7 3.20e+02 - 1.00e+00 1.00e+00h 1
41 -1.2624828e+01 6.53e-05 1.17e-09 -5.7 1.00e+01 - 1.00e+00 1.00e+00h 1
42 -1.2624828e+01 1.89e-10 1.90e-11 -5.7 1.40e-02 - 1.00e+00 1.00e+00h 1
43 -1.2625975e+01 7.36e-02 9.08e-07 -8.6 1.84e+02 - 9.54e-01 9.46e-01f 1
44 -1.2626039e+01 4.59e-04 5.48e-09 -8.6 2.31e+01 - 1.00e+00 1.00e+00h 1
45 -1.2626040e+01 1.98e-09 6.18e-11 -8.6 5.79e-02 - 1.00e+00 1.00e+00h 1
Number of Iterations....: 45
(scaled) (unscaled)
Objective...............: -1.2626039562353837e+01 -1.2626039562353837e+01
Dual infeasibility......: 6.1759819480755596e-11 6.1759819480755596e-11
Constraint violation....: 1.1176692551792170e-09 1.9790604710578918e-09
Complementarity.........: 2.5059118530201231e-09 2.5059118530201231e-09
Overall NLP error.......: 2.5059118530201231e-09 2.5059118530201231e-09
Number of objective function evaluations = 54
Number of objective gradient evaluations = 46
Number of equality constraint evaluations = 55
Number of inequality constraint evaluations = 0
Number of equality constraint Jacobian evaluations = 46
Number of inequality constraint Jacobian evaluations = 0
Number of Lagrangian Hessian evaluations = 45
Total CPU secs in IPOPT (w/o function evaluations) = 0.905
Total CPU secs in NLP function evaluations = 0.009
EXIT: Optimal Solution Found.
# Extract and plot the results using our custom function
### BEGIN SOLUTION
dopt_pyomo_doe_results = extract_plot_results(None, TC_Lab_DoE_D.model.scenario_blocks[0])
### END SOLUTION
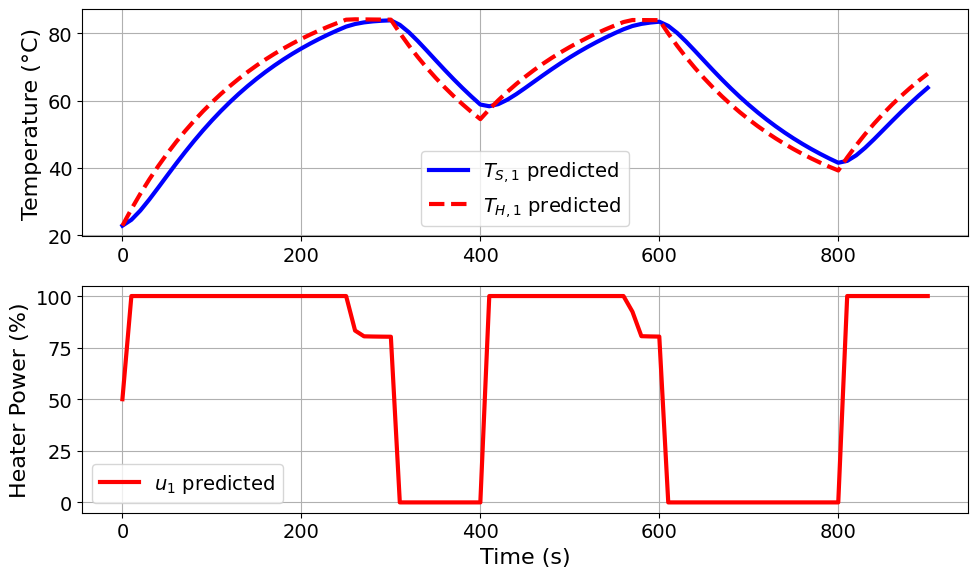
Model parameters:
Ua = 0.0421 Watts/degC
Ub = 0.0094 Watts/degC
CpH = 6.0274 Joules/degC
CpS = 0.1714 Joules/degC
# Compute the FIM at the optimal solution
### BEGIN SOLUTION
results_summary(TC_Lab_DoE_D.results['FIM'])
### END SOLUTION
======Results Summary======
Four design criteria log10() value:
A-optimality: 5.967636028118886
D-optimality: 12.62603956232522
E-optimality: -1.8550827141000377
Modified E-optimality: 7.772789117677075
FIM:
[[818563.0801634969, -620.1786056149823, -79956.24482342416, -3266.450673590944], [-620.1786056149823, 4866.710384232659, 15971.220200977912, 5229.79120056382], [-79956.24482342416, 15971.220200977912, 99087.96962372029, 18696.801379926546], [-3266.450673590944, 5229.79120056382, 18696.801379926546, 5670.408112132706]]
eigenvalues:
[8.27382639e+05 9.70342163e+04 3.77129903e+03 1.39610244e-02]
eigenvectors:
[[-9.93977609e-01 1.07151272e-01 -2.29590047e-02 4.07808937e-05]
[ 2.91390791e-03 1.76638285e-01 6.96995197e-01 -6.94973468e-01]
[ 1.09354088e-01 9.57308108e-01 -2.66548727e-01 -2.35509312e-02]
[ 6.45796596e-03 2.02184836e-01 6.65298697e-01 7.18649588e-01]]
Discussion: How do these results compare to our previous analysis?